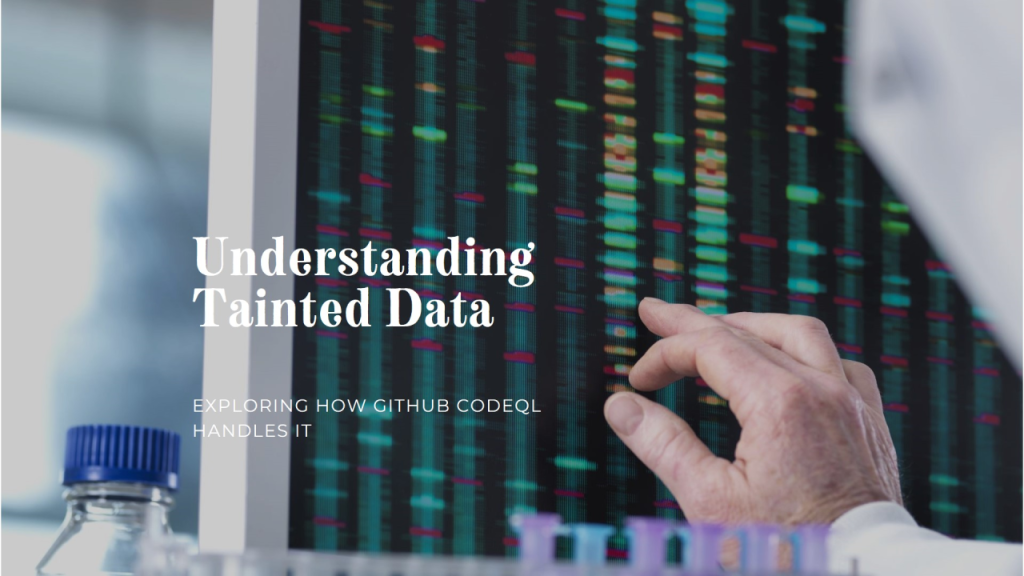
Ever wondered how untrusted inputs can become the Achilles’ heel of your application security? Today, we’re diving into a critical concept for developers and security professionals alike: tainted data. Understanding tainted data is key to preventing vulnerabilities like SQL Injection, Cross-Site Scripting (XSS), and others that can compromise the safety of your applications.
What is Tainted Data?
In the world of software security, tainted data refers to any input that comes from an external, untrusted source. Examples of such sources include:
- User Inputs: Form fields, URL parameters, or uploaded files.
- External APIs: Data coming from a third-party service.
- Environment Variables: Particularly when those variables can be set externally.
Tainted data is dangerous because it may carry malicious payloads intended to exploit vulnerabilities in your application. Until it is validated or sanitized, it is considered untrustworthy.
Take the following example in Java:
String userInput = request.getParameter("username");
response.getWriter().println("<h1>Welcome, " + userInput + "</h1>");
In this case, userInput
is tainted because it originates directly from the user and is inserted into the HTML output without any sanitization. If an attacker submits a script as the username, it could be executed in the browser, resulting in an XSS vulnerability.
Why is Tainted Data Dangerous?
Tainted data becomes a significant risk when it reaches a sensitive “sink” without being properly handled. A sink is any part of the code where the tainted data could do harm, such as being used in a database query, an HTML response, or file system operations.
Here are some common vulnerabilities associated with tainted data:
- SQL Injection: Occurs when user inputs are concatenated into an SQL query without proper escaping or parameterization. An attacker could inject malicious SQL commands that might alter your database in unintended ways.
String query = "SELECT * FROM users WHERE username = '" + userInput + "'";
// This is vulnerable to SQL Injection if userInput contains malicious SQL code.
- Cross-Site Scripting (XSS): Occurs when unsanitized user input is directly reflected in the webpage output. This allows attackers to inject and execute JavaScript in the context of another user, potentially stealing their data.
- Path Traversal: Tainted data used in file paths could allow attackers to navigate outside of allowed directories, potentially accessing sensitive files on the server.
How to Manage Tainted Data Effectively
The best way to avoid the dangers of tainted data is to implement security measures at every step of data handling:
- Input Validation: Validate incoming data to ensure it meets expected formats and types. For instance, if you expect an email address, ensure the input matches a valid email pattern.
if (!userInput.matches("^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$")) {
throw new IllegalArgumentException("Invalid email address");
}
- Sanitization and Escaping: Modify data to ensure it is safe before it reaches critical points in your application. For SQL queries, always use parameterized queries instead of string concatenation.
String query = "SELECT * FROM users WHERE username = ?";
PreparedStatement stmt = connection.prepareStatement(query);
stmt.setString(1, userInput); // Parameterized to avoid SQL Injection
For HTML output, use libraries that automatically escape HTML characters to prevent XSS.
- Taint Tracking: Use static analysis tools like CodeQL or Fortify to automatically detect flows of tainted data. These tools can identify whether untrusted inputs reach sensitive sinks without adequate sanitization.
Taint Tracking with CodeQL
Taint tracking is a technique used by static analysis tools to determine the flow of tainted data through your codebase. It helps you understand if user input, which starts out as tainted, reaches a sensitive area without proper validation or cleaning. This helps prevent a variety of injection vulnerabilities.
- Sources: In the context of taint tracking, a source is any point where potentially tainted data enters your system, such as user inputs (
request.getParameter()
in a servlet). - Sinks: A sink is any place where using tainted data without sanitization could cause harm, like an SQL execution (
executeQuery()
) or HTML response. - Sanitizers: These are points in the code where data is cleaned or verified before further use. Proper use of sanitizers ensures tainted data cannot reach a sink and cause vulnerabilities.
Example Scenario: SQL Injection
Let’s consider a scenario where a developer uses user input to construct a query:
String userInput = request.getParameter("id");
String query = "SELECT * FROM users WHERE id = " + userInput;
In this case, if userInput
contains malicious SQL code (e.g., 1 OR 1=1
), it can alter the query and return unintended results, or worse, compromise the database.
To fix this, parameterized queries are used:
String query = "SELECT * FROM users WHERE id = ?";
PreparedStatement stmt = connection.prepareStatement(query);
stmt.setString(1, userInput);
This way, the database treats userInput
as a value rather than as part of the SQL command, preventing injection attacks.
Conclusion
Tainted data can pose significant security threats if not managed properly. By understanding the risks associated with tainted data and employing techniques like input validation, sanitization, and taint tracking, you can build more secure applications.
Modern security tools like CodeQL offer advanced taint tracking capabilities that help developers identify vulnerabilities in the codebase before they become real issues. Implementing these best practices can save your organization from a wide range of common, yet dangerous, security threats.
How are you handling tainted data in your projects? Are you using static analysis tools like CodeQL or Fortify to track data flows and secure your applications? Let’s discuss more about securing our codebases and making the web a safer place.